Слайд 2Метод Union
public static System.Collections.Generic.IEnumerable Union (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second);
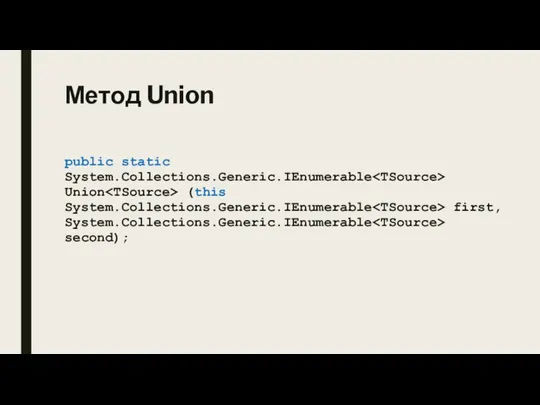
Слайд 3Метод Union
int[] ints1 = { 5, 3, 9, 7, 5, 9, 3,
![Метод Union int[] ints1 = { 5, 3, 9, 7, 5, 9,](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-2.jpg)
7 };
int[] ints2 = { 8, 3, 6, 4, 4, 9, 1, 0 };
IEnumerable union = ints1.Union(ints2);
foreach (int num in union)
{
Console.Write("{0} ", num);
}
Слайд 4Метод Union
public bool Equals(ProductA other)
{
if (other is null)
return false;
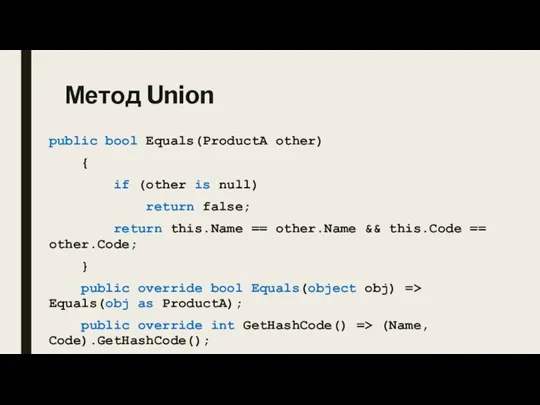
return this.Name == other.Name && this.Code == other.Code;
}
public override bool Equals(object obj) => Equals(obj as ProductA);
public override int GetHashCode() => (Name, Code).GetHashCode();
Слайд 5Метод Union
IEnumerable union =
store1.Union(store2);
foreach (var product in union)
Console.WriteLine(product.Name + "
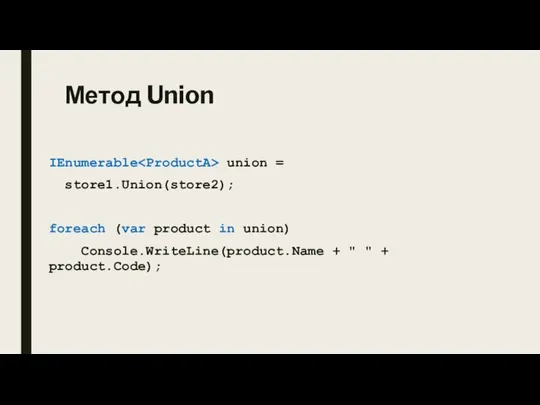
" + product.Code);
Слайд 6Метод Intersect
public static System.Collections.Generic.IEnumerable Intersect (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second, System.Collections.Generic.IEqualityComparer comparer);
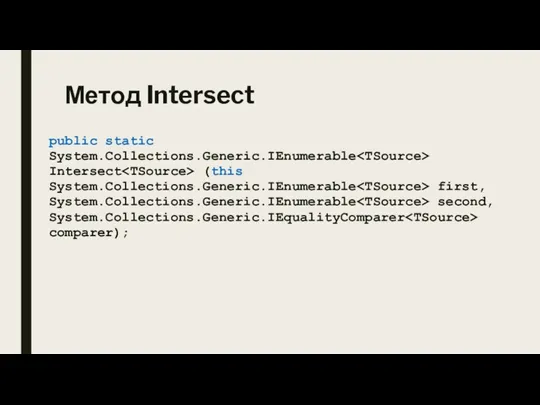
Слайд 7Метод Intersect
public static System.Collections.Generic.IEnumerable Intersect (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second);
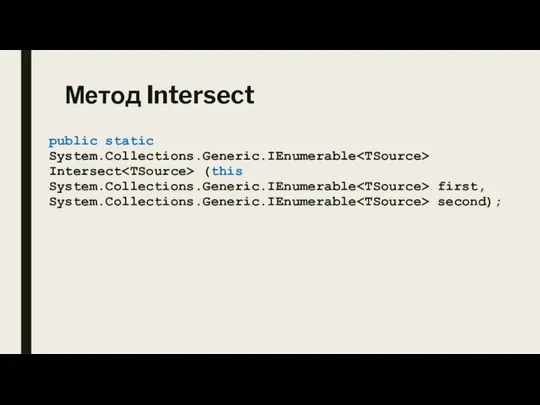
Слайд 8Метод Except
public static System.Collections.Generic.IEnumerable Except (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second);
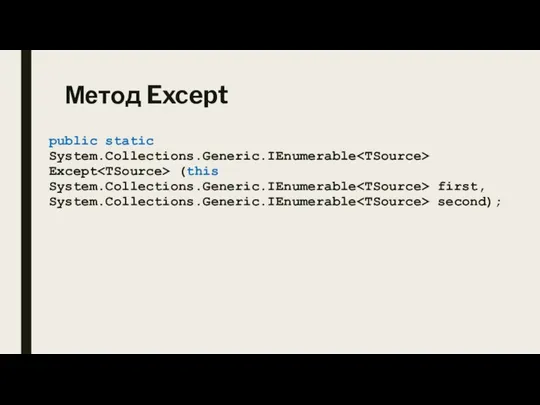
Слайд 9Метод Except
double[] numbers1 = { 2.0, 2.0, 2.1, 2.2, 2.3, 2.3, 2.4,
![Метод Except double[] numbers1 = { 2.0, 2.0, 2.1, 2.2, 2.3, 2.3,](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-8.jpg)
2.5 };
double[] numbers2 = { 2.2 };
IEnumerable onlyInFirstSet = numbers1.Except(numbers2);
foreach (double number in onlyInFirstSet)
Console.WriteLine(number);
Слайд 10Метод Except
public static System.Collections.Generic.IEnumerable Except (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second, System.Collections.Generic.IEqualityComparer comparer);
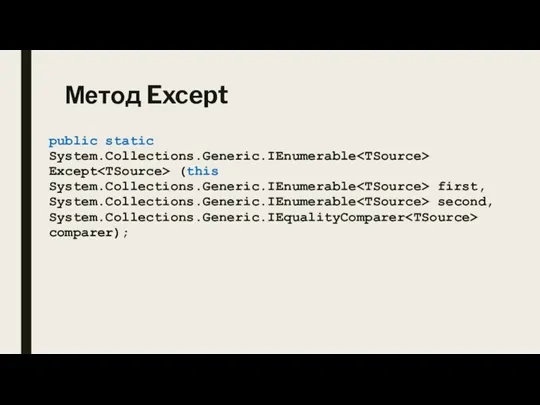
Слайд 11Метод Contains
public static bool Contains (this System.Collections.Generic.IEnumerable source, TSource value);
public static bool
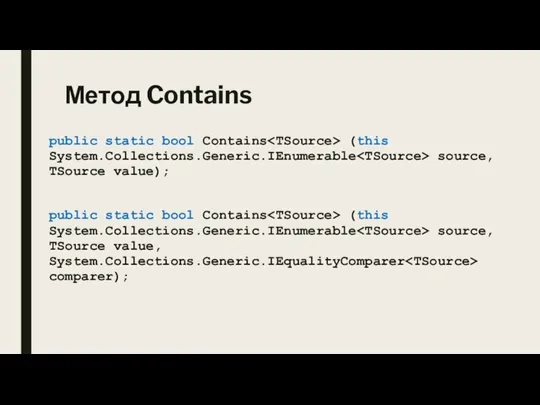
Contains (this System.Collections.Generic.IEnumerable source, TSource value, System.Collections.Generic.IEqualityComparer comparer);
Слайд 12Метод Single
public static TSource Single (this System.Collections.Generic.IEnumerable source, Func predicate);
public static TSource
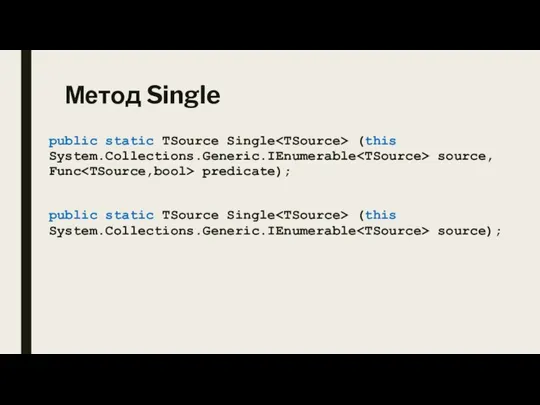
Single (this System.Collections.Generic.IEnumerable source);
Слайд 13Метод Single
string[] fruits = { "apple", "banana", "mango",
"orange", "passionfruit", "grape" };
string
![Метод Single string[] fruits = { "apple", "banana", "mango", "orange", "passionfruit", "grape"](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-12.jpg)
fruit1 = fruits.Single(fruit => fruit.Length > 10);
Console.WriteLine(fruit1);
Слайд 14Метод Single
string[] fruits1 = { "orange" };
string fruit1 = fruits1.Single();
Console.WriteLine(fruit1);
![Метод Single string[] fruits1 = { "orange" }; string fruit1 = fruits1.Single(); Console.WriteLine(fruit1);](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-13.jpg)
Слайд 15Метод SingleOrDefault
public static TSource SingleOrDefault (this System.Collections.Generic.IEnumerable source);
public static TSource SingleOrDefault (this
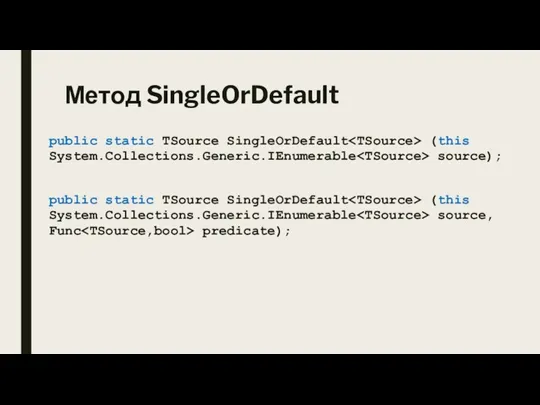
System.Collections.Generic.IEnumerable source, Func predicate);
Слайд 16Метод Any
public static bool Any (this System.Collections.Generic.IEnumerable source);
List numbers = new List
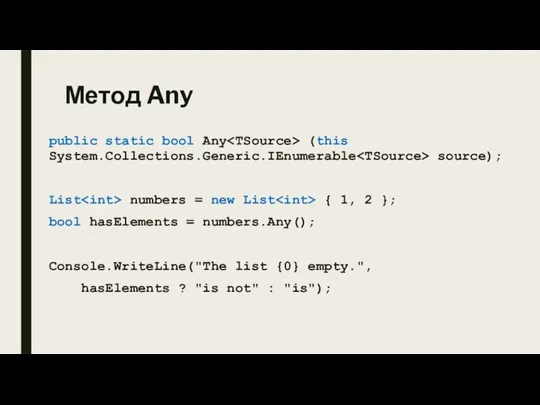
{ 1, 2 };
bool hasElements = numbers.Any();
Console.WriteLine("The list {0} empty.",
hasElements ? "is not" : "is");
Слайд 17Метод Any
public static bool Any (this System.Collections.Generic.IEnumerable source, Func predicate);
Pet[] pets =
![Метод Any public static bool Any (this System.Collections.Generic.IEnumerable source, Func predicate); Pet[]](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-16.jpg)
{
new Pet { Name="Barley", Age=8, Vaccinated=true },
new Pet { Name="Boots", Age=4, Vaccinated=false },
new Pet { Name="Whiskers", Age=1, Vaccinated=false }
};
bool unvaccinated = pets.Any(p => p.Age > 1 && p.Vaccinated == false);
Слайд 18Метод All
public static bool All (this System.Collections.Generic.IEnumerable source, Func predicate);
Pet[] pets =
![Метод All public static bool All (this System.Collections.Generic.IEnumerable source, Func predicate); Pet[]](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-17.jpg)
{
new Pet { Name="Barley", Age=10 },
new Pet { Name="Boots", Age=4 },
new Pet { Name="Whiskers", Age=6 } };
bool allStartWithB = pets.All(pet => pet.Name.StartsWith("B"));
Слайд 19Метод SequenceEqual
public static bool SequenceEqual (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second);
List pets1 =
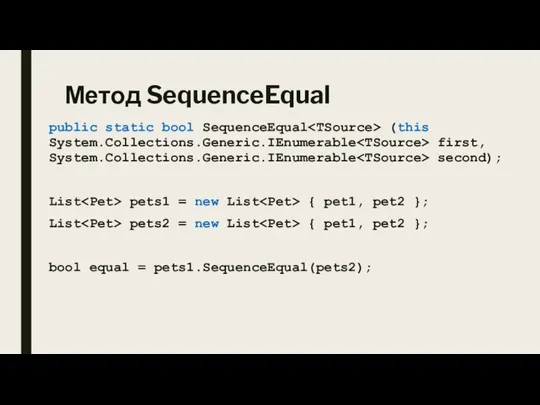
new List
{ pet1, pet2 };
List pets2 = new List { pet1, pet2 };
bool equal = pets1.SequenceEqual(pets2);
Слайд 20Метод SequenceEqual
public static bool SequenceEqual (this System.Collections.Generic.IEnumerable first, System.Collections.Generic.IEnumerable second, System.Collections.Generic.IEqualityComparer comparer);
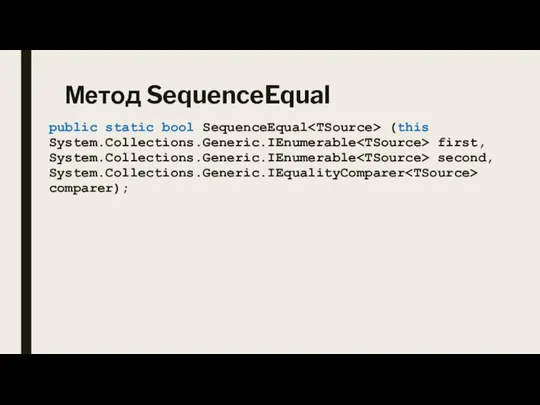
Слайд 21Метод SequenceEqual
class ProductComparer : IEqualityComparer
{
public bool Equals(Product x, Product y)
{
if
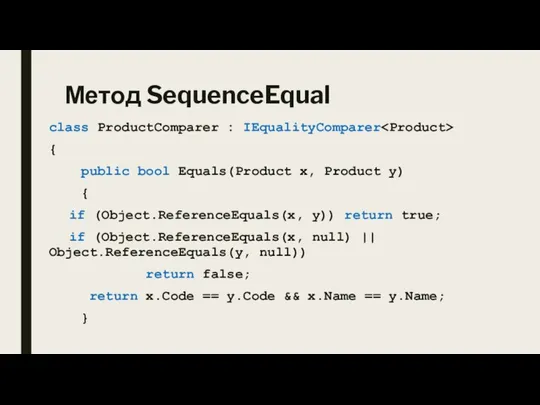
(Object.ReferenceEquals(x, y)) return true;
if (Object.ReferenceEquals(x, null) || Object.ReferenceEquals(y, null))
return false;
return x.Code == y.Code && x.Name == y.Name;
}
Слайд 22Метод SequenceEqual (продолжение)
public int GetHashCode(Product product)
{
if (Object.ReferenceEquals(product, null)) return 0;
int
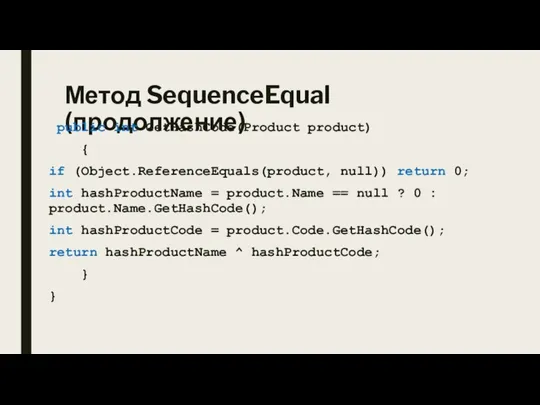
hashProductName = product.Name == null ? 0 : product.Name.GetHashCode();
int hashProductCode = product.Code.GetHashCode();
return hashProductName ^ hashProductCode;
}
}
Слайд 23Метод OfType
public static System.Collections.Generic.IEnumerable OfType (this System.Collections.IEnumerable source);
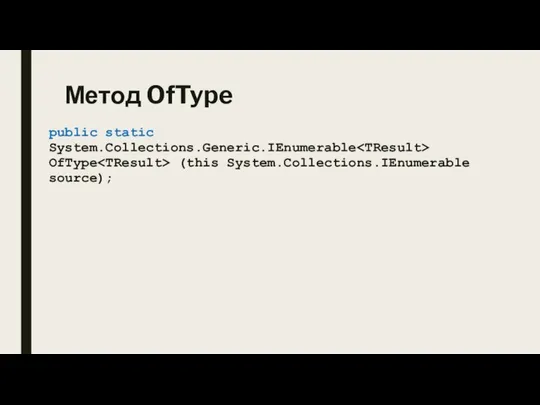
Слайд 24Метод OfType
System.Collections.ArrayList fruits = new System.Collections.ArrayList(4);
fruits.Add("Mango");fruits.Add("Orange");
fruits.Add("Apple");fruits.Add(3.0);fruits.Add("Banana");
IEnumerable query1 = fruits.OfType();
Console.WriteLine("Elements of type 'string'
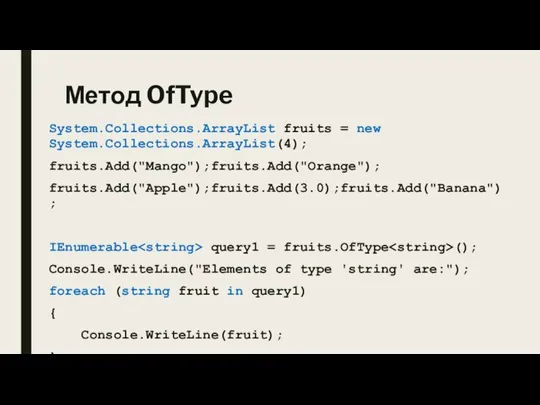
are:");
foreach (string fruit in query1)
{
Console.WriteLine(fruit);
}
Слайд 25Метод Cast
public static System.Collections.Generic.IEnumerable Cast (this System.Collections.IEnumerable source);
System.Collections.ArrayList fruits = new System.Collections.ArrayList();
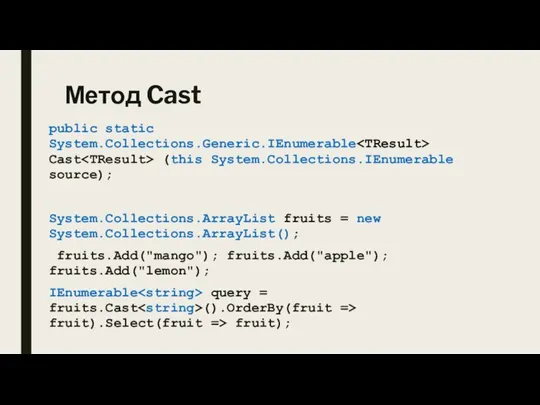
fruits.Add("mango"); fruits.Add("apple"); fruits.Add("lemon");
IEnumerable query = fruits.Cast().OrderBy(fruit => fruit).Select(fruit => fruit);
Слайд 26Метод перевода
public static TSource[] ToArray (…);
public static System.Collections.Generic.Dictionary ToDictionary (…);
public static System.Collections.Generic.List
![Метод перевода public static TSource[] ToArray (…); public static System.Collections.Generic.Dictionary ToDictionary (…);](/_ipx/f_webp&q_80&fit_contain&s_1440x1080/imagesDir/jpg/1182097/slide-25.jpg)
ToList (…);
public static System.Collections.Generic.HashSet ToHashSet (…);
Слайд 27Метод Aggregate
public static TAccumulate Aggregate (this System.Collections.Generic.IEnumerable source, TAccumulate seed, Func func);
int[]
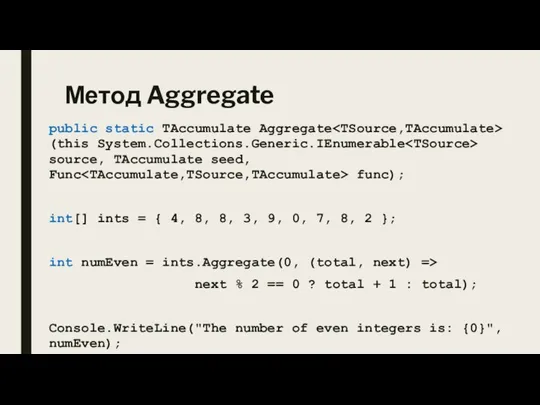
ints = { 4, 8, 8, 3, 9, 0, 7, 8, 2 };
int numEven = ints.Aggregate(0, (total, next) =>
next % 2 == 0 ? total + 1 : total);
Console.WriteLine("The number of even integers is: {0}", numEven);
Слайд 28Метод Join
public static System.Collections.Generic.IEnumerable Join (this System.Collections.Generic.IEnumerable outer, System.Collections.Generic.IEnumerable inner, Func outerKeySelector,
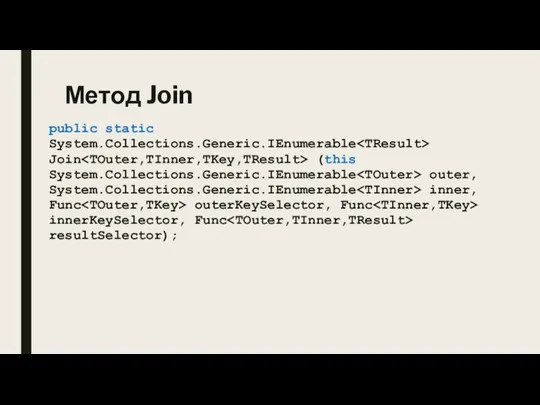
Func innerKeySelector, Func resultSelector);
Слайд 29Метод Join
List people = new List { magnus, terry, charlotte };
List
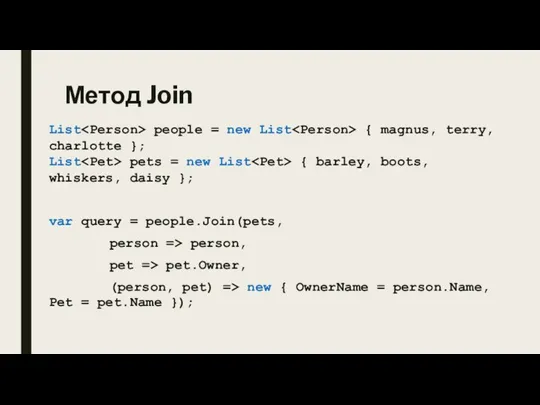
pets = new List
{ barley, boots, whiskers, daisy };
var query = people.Join(pets,
person => person,
pet => pet.Owner,
(person, pet) => new { OwnerName = person.Name, Pet = pet.Name });
Слайд 30Метод GroupJoin
public static System.Collections.Generic.IEnumerable GroupJoin (this System.Collections.Generic.IEnumerable outer, System.Collections.Generic.IEnumerable inner, Func outerKeySelector,
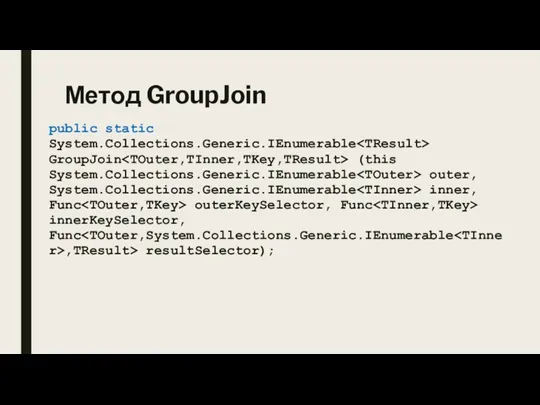
Func innerKeySelector, Func,TResult> resultSelector);
Слайд 31Метод GroupJoin
List people = new List { magnus, terry, charlotte };
List
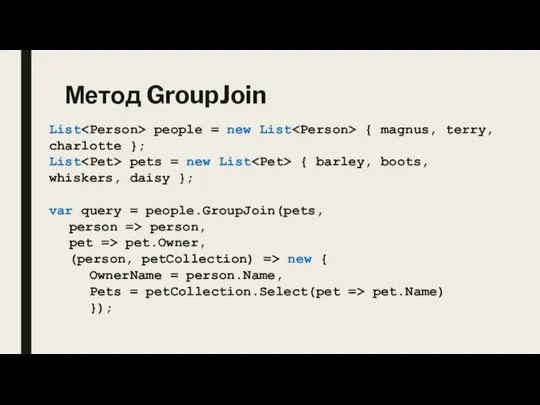
pets = new List
{ barley, boots, whiskers, daisy };
var query = people.GroupJoin(pets,
person => person,
pet => pet.Owner,
(person, petCollection) => new {
OwnerName = person.Name,
Pets = petCollection.Select(pet => pet.Name) });
Слайд 32Метод GroupBy
public static System.Collections.Generic.IEnumerable> GroupBy (this System.Collections.Generic.IEnumerable source, Func keySelector, Func elementSelector);
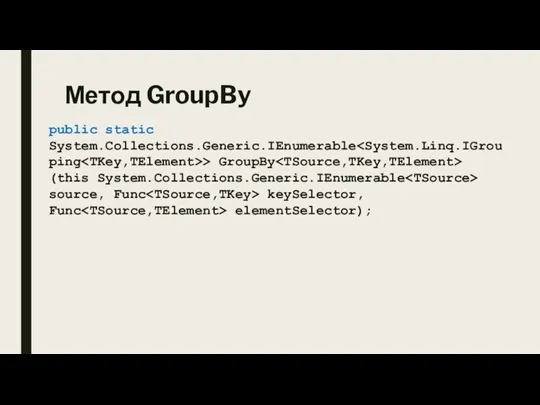