Слайд 2What should I know about GIT?
Source control
Branching
Merge
Rebase
Cherry pick
Stash
Tag
Etc.
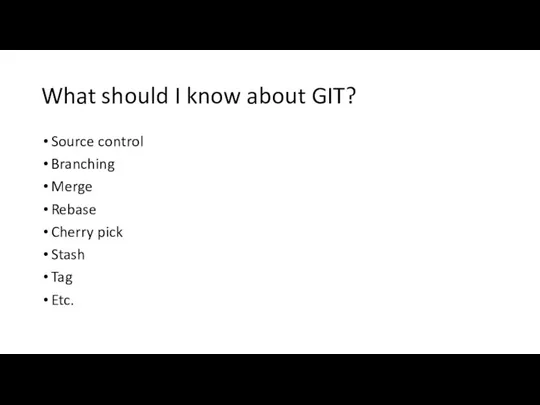
Слайд 4Why I should use Typescript?
Less bugs
Development performance
Features: type checking, autocompletion, source documentation
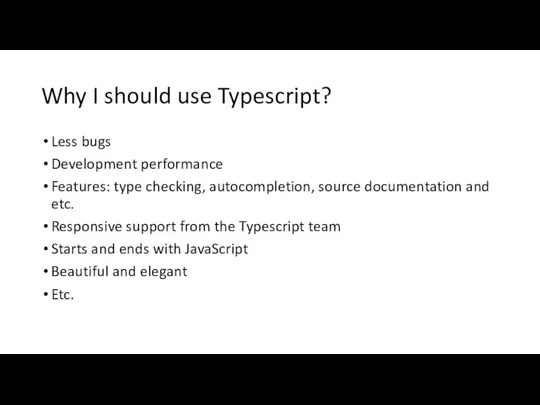
and etc.
Responsive support from the Typescript team
Starts and ends with JavaScript
Beautiful and elegant
Etc.
Слайд 5Getting started
https://nodejs.org/en/
npm install -g typescript (or install locally in dev dependencies)
tsc helloworld.ts
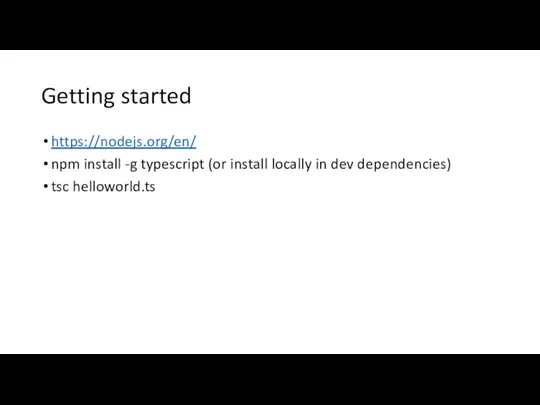
Слайд 6How to compile
tsc helloworld.ts
tsconfig.json
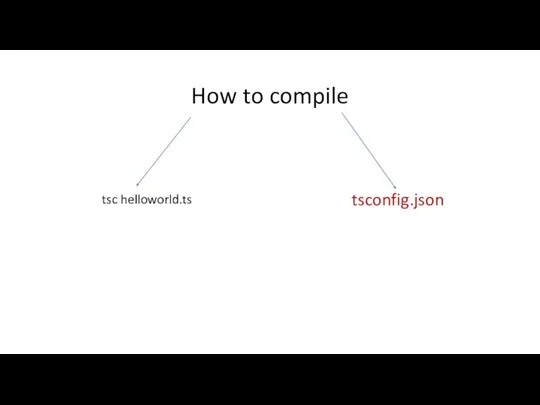
Слайд 7Settings
target
removeComments
outDir
sourceMap
outFile
files
Etc.
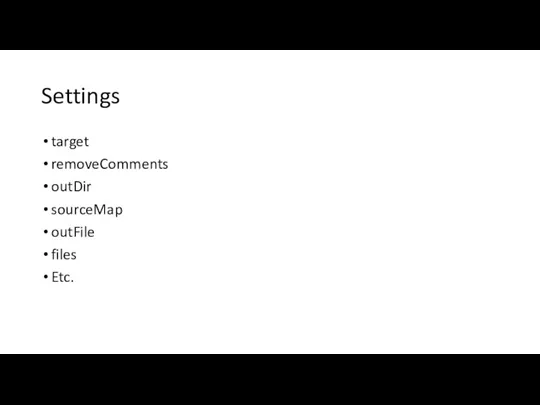
Слайд 8Variables
Use ES6 let and const to declare variables in Typescript.
let user: User
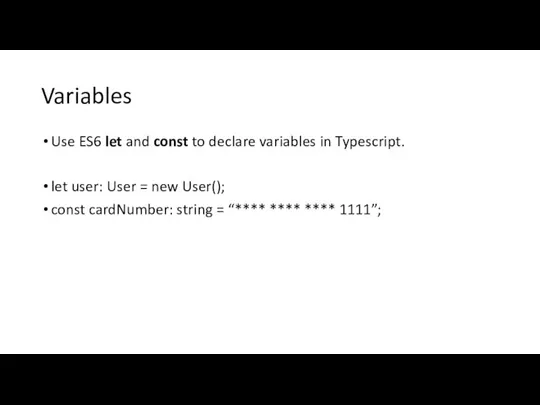
= new User();
const cardNumber: string = “**** **** **** 1111”;
Слайд 9Types
boolean
number
String
Object
Array
Tuple
enum
null
undefined
void
never
any
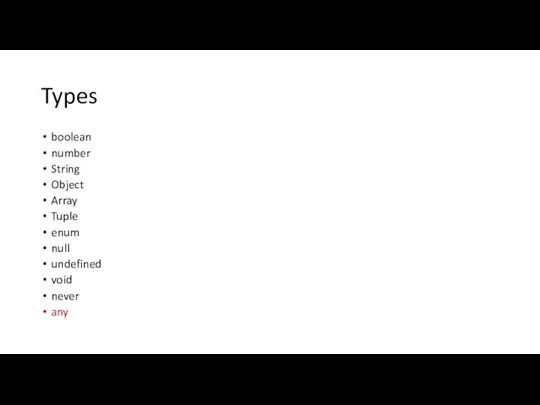
Слайд 10Type declaration
declare type primitive = number | string | boolean| null |
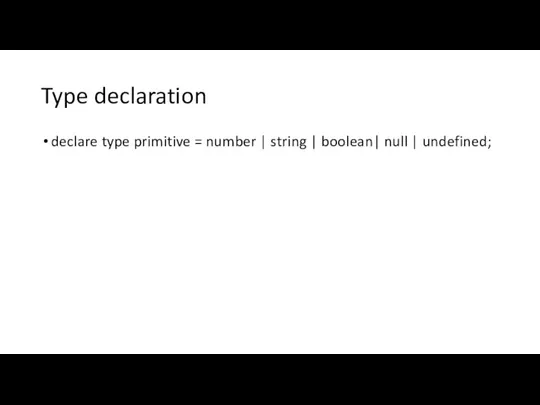
undefined;
Слайд 12What should I know about classes in TS?
fields
methods
constructor
static fields and methods
private/public/protected
get/set
readonly
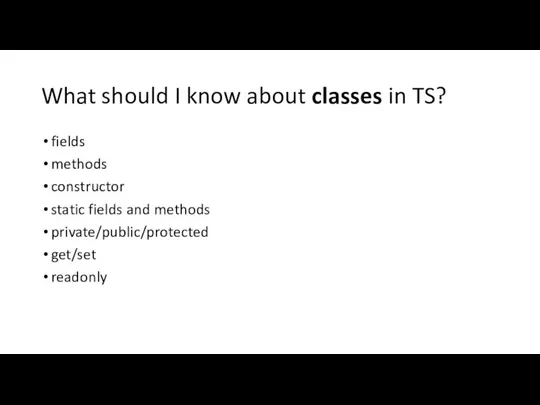
Слайд 13Inheritance and abstract classes
basic inheritance mechanism in general the same as in
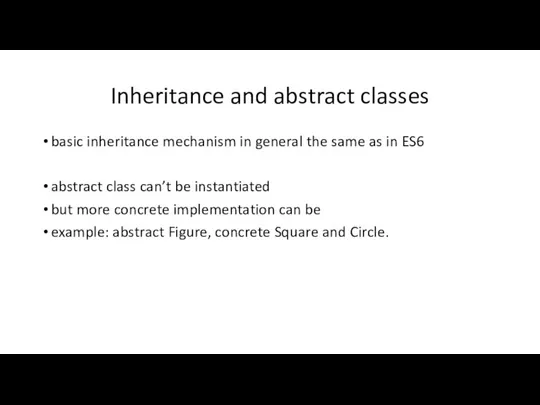
ES6
abstract class can’t be instantiated
but more concrete implementation can be
example: abstract Figure, concrete Square and Circle.
Слайд 14What should I know about interfaces in TS?
syntax
optional and readonly properties
implementation
Interface inheritance
Function
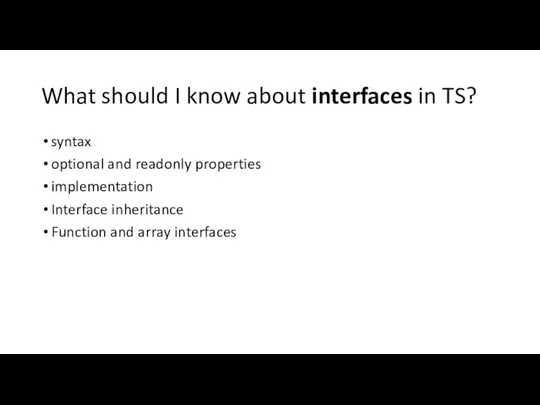
and array interfaces
Слайд 15Generics
Use generics when something should work with any data types.
You can use
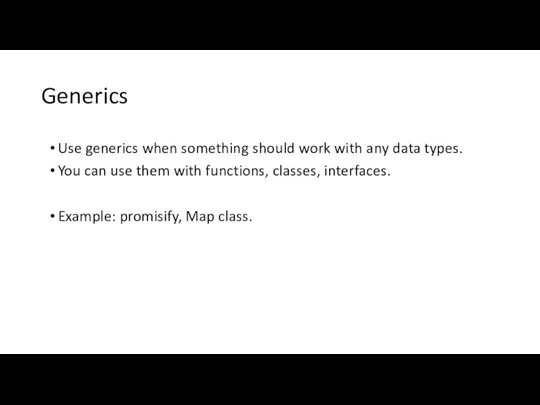
them with functions, classes, interfaces.
Example: promisify, Map class.
Слайд 16Namespaces
Namespaces contains a group of classes, functions, interfaces, variables, other namespaces, etc.
Use
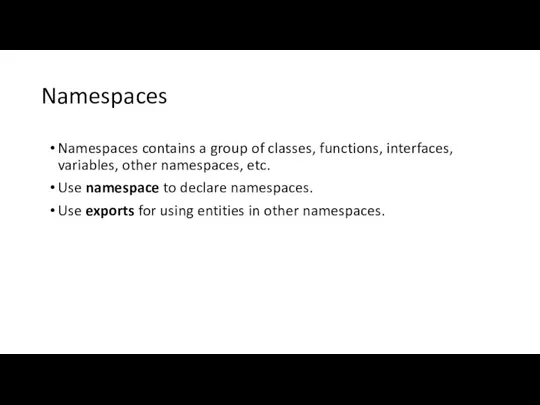
namespace to declare namespaces.
Use exports for using entities in other namespaces.
Слайд 17Modules
TS support the following modules:
AMD (Asynchronys Module Defenition)
CommonJS
UMD (Universal Module Defenition)
System
ES 2015
Use
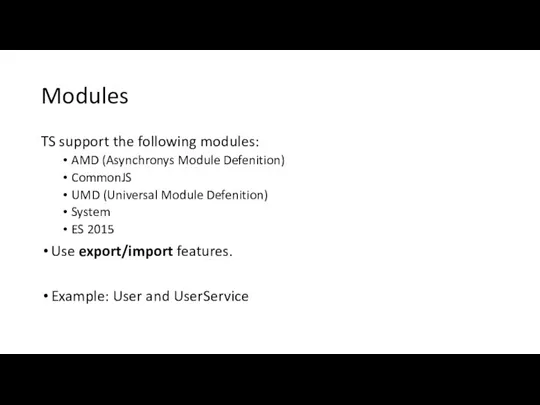
export/import features.
Example: User and UserService
Слайд 18Decorators
Allow to add metadata to classes or their members for changing their
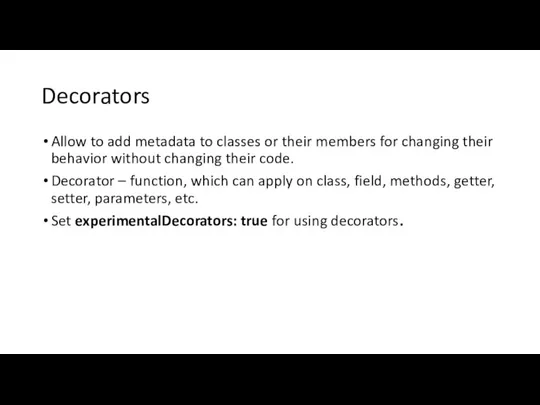
behavior without changing their code.
Decorator – function, which can apply on class, field, methods, getter, setter, parameters, etc.
Set experimentalDecorators: true for using decorators.