- Главная
- Информатика
- Binding event handlers
Содержание
- 2. THIS IN EVENT HANDLERS When you define a component using an ES6 class, a common pattern
- 3. BINDING IN RENDER We can bind in render method. The problem with this syntax is that
- 4. ARROW FUNCTIOB IN RENDER We can create an arrow function in render method. The problem with
- 5. BINDING IN CONSTRUCTOR Binding event handler in constructor is considered to be a good practice. export
- 6. PUBLIC CLASS FIELDS SYNTAX Defining event handler as a public class field is also considered to
- 8. Скачать презентацию
Слайд 2THIS IN EVENT HANDLERS
When you define a component using an ES6 class, a
THIS IN EVENT HANDLERS
When you define a component using an ES6 class, a
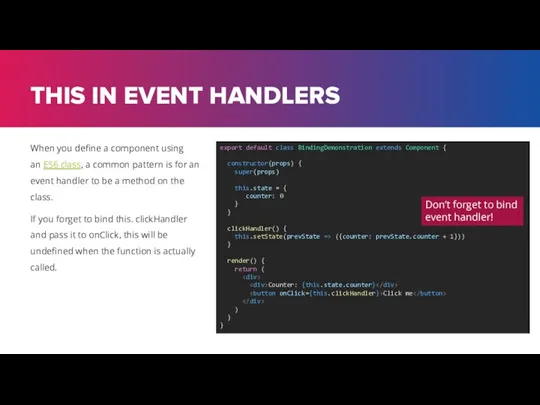
If you forget to bind this. clickHandler and pass it to onClick, this will be undefined when the function is actually called.
export default class BindingDemonstration extends Component {
constructor(props) {
super(props)
this.state = {
counter: 0
}
}
clickHandler() {
this.setState(prevState => ({counter: prevState.counter + 1}))
}
render() {
return (
)
}
}
Don’t forget to bind event handler!
Слайд 3BINDING IN RENDER
We can bind in render method.
The problem with this syntax
BINDING IN RENDER
We can bind in render method.
The problem with this syntax
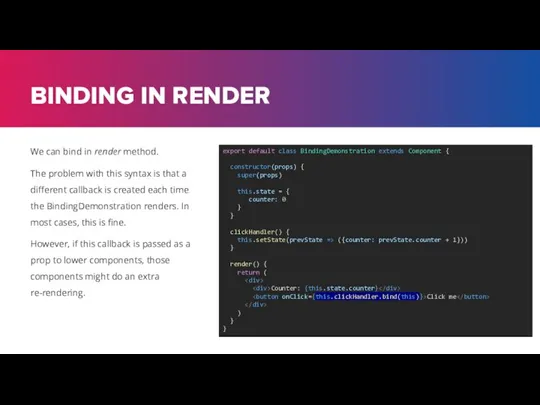
However, if this callback is passed as a prop to lower components, those components might do an extra re-rendering.
export default class BindingDemonstration extends Component {
constructor(props) {
super(props)
this.state = {
counter: 0
}
}
clickHandler() {
this.setState(prevState => ({counter: prevState.counter + 1}))
}
render() {
return (
)
}
}
Слайд 4ARROW FUNCTIOB IN RENDER
We can create an arrow function in render method.
The
ARROW FUNCTIOB IN RENDER
We can create an arrow function in render method.
The
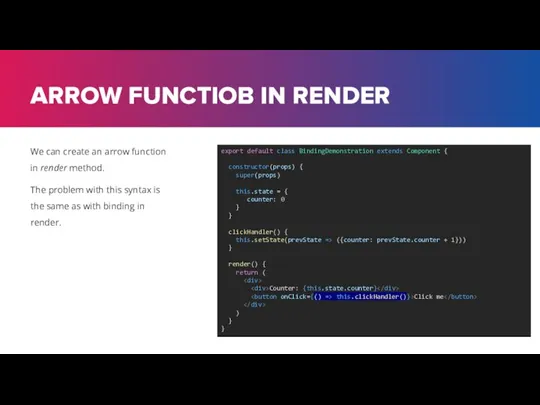
export default class BindingDemonstration extends Component {
constructor(props) {
super(props)
this.state = {
counter: 0
}
}
clickHandler() {
this.setState(prevState => ({counter: prevState.counter + 1}))
}
render() {
return (
)
}
}
Слайд 5BINDING IN CONSTRUCTOR
Binding event handler in constructor is considered to be a
BINDING IN CONSTRUCTOR
Binding event handler in constructor is considered to be a
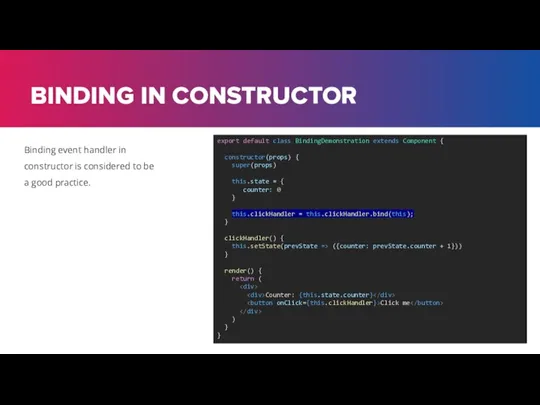
export default class BindingDemonstration extends Component {
constructor(props) {
super(props)
this.state = {
counter: 0
}
this.clickHandler = this.clickHandler.bind(this);
}
clickHandler() {
this.setState(prevState => ({counter: prevState.counter + 1}))
}
render() {
return (
)
}
}
Слайд 6PUBLIC CLASS FIELDS SYNTAX
Defining event handler as a public class field is
PUBLIC CLASS FIELDS SYNTAX
Defining event handler as a public class field is
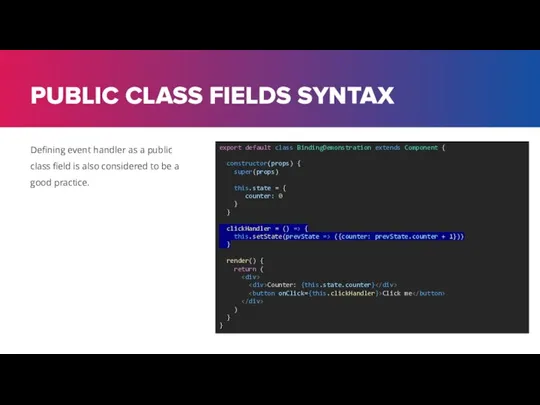
export default class BindingDemonstration extends Component {
constructor(props) {
super(props)
this.state = {
counter: 0
}
}
clickHandler = () => {
this.setState(prevState => ({counter: prevState.counter + 1}))
}
render() {
return (
)
}
}